Design patterns are guidelines for solving repetitive problems.
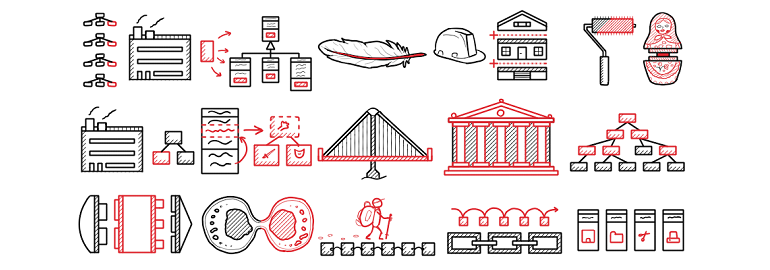
Quote from Wikipedia:
Software design pattern is a general, reusable solution to a commonly occurring problem within a given context in software design.
Types of Design Patterns
- Database Patterns
- Generating Objects
- Patterns for Flexible Object Programming
- Performing and Representing Tasks
- Enterprise Patterns
Data Mapper
The data mapper pattern is an architectural pattern. It was named by Martin Fowler in his 2003 book Patterns of Enterprise Application Architecture. The interface of an object conforming to this pattern would include functions such as Create, Read, Update, and Delete, that operate on objects that represent domain entity types in a data store.
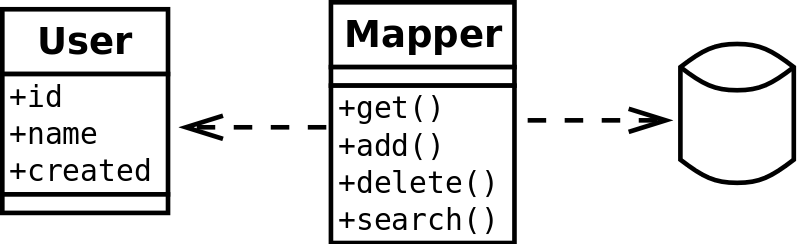
Purpose
Create specialist classes for mapping Domain Model objects to and from relational databases.
Example
<?php
class NewsMapper
{
....
public function save(News $news) { ... }
public function getById($id) { ... }
public function findLatestNews() { ... }
}
$mapper = new NewsMapper($pdo);
$someNews = $mapper->getById(10);
$someNews->title = 'New title';
$mapper->save($someNews);
$newNews = new News();
$newNews->title = 'News title!';
$newNews->text = 'Text of news';
$mapper->save($newNews);
Identity Map
The identity map pattern is a database access design pattern used to improve performance by providing a context-specific, in-memory cache to prevent duplicate retrieval of the same object data from the database.
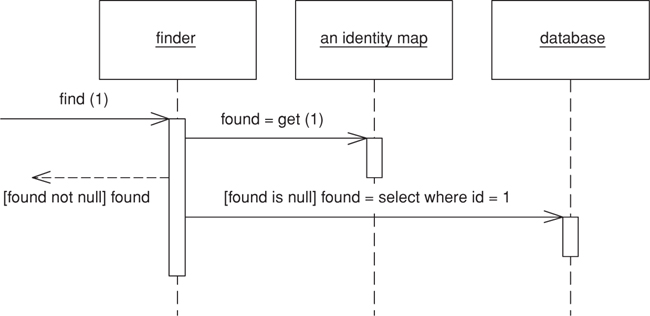
Purpose
Keep track of all the objects in your system to prevent duplicate instantiations and unnecessary trips to the database.
Example
By using Data-Mapper pattern without an identity map, you can easily run into problems because you may have more than one object that references the same domain entity. The identity map solves this problem by acting as a registry for all loaded domain instances.
$userMapper = new UserMapper($pdo);
$user1 = $userMapper->find(1); // creates new object
$user2 = $userMapper->find(1); // returns same object
echo $user1->getNickname(); // joe123
echo $user2->getNickname(); // joe123
$user1->setNickname('bob78');
echo $user1->getNickname(); // bob78
echo $user2->getNickname(); // bob78 -> yes, much better
Unit of Work
The Unit of Work pattern is used to group one or more operations (usually database operations) into a single transaction or “unit of work”, so that all operations either pass or fail as one.
Purpose
Automate the process by which objects are saved to the database, ensuring that only objects that have been changed are updated, and only those that have been newly created are inserted.
Example
A lightweight interface of a UOW might look like this:
<?php
namespace ModelRepository;
use ModelEntityInterface;
interface UnitOfWorkInterface
{
public function fetchById($id);
public function registerNew(EntityInterface $entity);
public function registerClean(EntityInterface $entity);
public function registerDirty(EntityInterface $entity);
public function registerDeleted(EntityInterface $entity);
public function commit();
public function rollback();
public function clear();
}
Lazy Load
Lazy loading is a design pattern commonly used in computer programming to defer initialization of an object until the point at which it is needed. It can contribute to efficiency in the program's operation if properly and appropriately used. The opposite of lazy loading is eager loading.
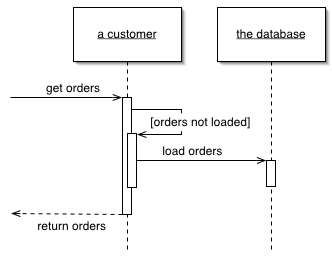
Purpose
Defer object creation, and even database queries, until they are actually needed.
Domain Object Factory
Lazy loading is a design pattern commonly used in computer programming to defer initialization of an object until the point at which it is needed. It can contribute to efficiency in the program's operation if properly and appropriately used. The opposite of lazy loading is eager loading.
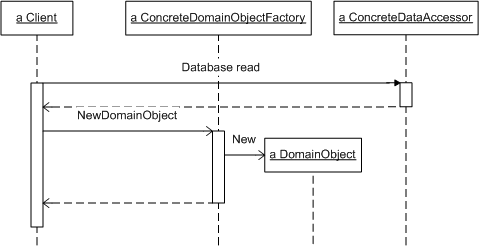
Purpose
Populates domain objects based on query results.
Identity Object
Object identity is a fundamental object orientation concept. With object identity, objects can contain or refer to other objects. Identity is a property of an object that distinguishes the object from all other objects in the application.
Purpose
Encapsulate the logic for constructing SQL queries. Allow clients to construct query criteria without reference to the underlying database.
Domain Object Assembler
Domain Object Assembler constructs a controller that manages the high-level process of data storage and retrieval.
Purpose
Populates, persists, and deletes domain objects using a uniform factory framework.
Summary
In this post, we looked at the following database patterns:
- Data Mapper
- Identity map
- Unit of Work
- Lazy Load
- Domain Object Factory
- Identity Object
- Domain Object Assembler
Marat Badykov
Full-stack web developer. I enjoy writing Php, Java, and Js.