Design patterns are guidelines for solving repetitive problems.
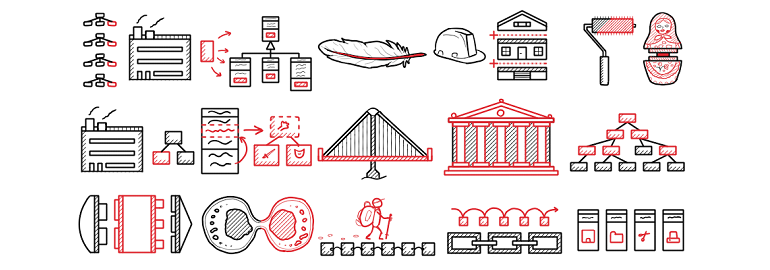
Quote from Wikipedia:
Software design pattern is a general, reusable solution to a commonly occurring problem within a given context in software design.
Types of Design Patterns
- Patterns for Flexible Object Programming
- Generating Objects
- Performing and Representing Tasks
- Enterprise Patterns
- Database Patterns
The Composite Pattern
The composite pattern describes a group of objects that is treated the same way as a single instance of the same type of object. The intent of a composite is to "compose" objects into tree structures to represent part-whole hierarchies. Implementing the composite pattern lets clients treat individual objects and compositions uniformly.
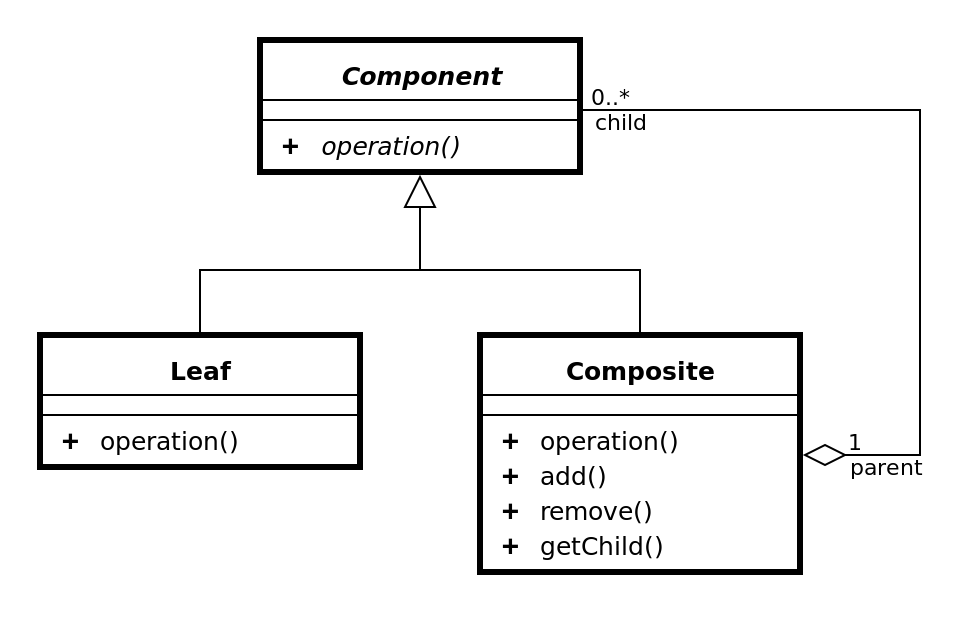
Purpose
Composing structures in which groups of objects can be used as if they were individual objects.
Implementation
class ComponentException extends Exception {};
abstract class Component
{
protected $_children = array();
abstract public function Add(Component $Component);
abstract public function Remove($index);
abstract public function GetChild($index);
abstract public function GetChildren();
abstract public function Operation();
}
class Composite extends Component
{
public function Add(Component $Component)
{
$this -> _children[] = $Component;
}
public function GetChild($index)
{
if (! isset($this -> _children[$index]))
{
throw new ComponentException("Child not exists");
}
return $this -> _children[$index];
}
public function Operation()
{
print "I am composite. I have " . count($this -> GetChildren()) . " children\n";
foreach($this -> GetChildren() as $Child)
{
$Child -> Operation();
}
}
public function Remove($index)
{
if (! isset($this -> _children[$index]))
{
throw new ComponentException("Child not exists");
}
unset($this -> _children[$index]);
}
public function GetChildren()
{
return $this -> _children;
}
}
class Leaf extends Component
{
public function Add(Component $Component)
{
throw new ComponentException("I can't append child to myself");
}
public function GetChild($index)
{
throw new ComponentException("Child not exists");
}
public function Operation()
{
print "I am leaf\n";
}
public function Remove($index)
{
throw new ComponentException("Child not exists");
}
public function GetChildren()
{
return array();
}
}
The Decorator Pattern
The decorator pattern is a design pattern that allows behavior to be added to an individual object, dynamically, without affecting the behavior of other objects from the same class.
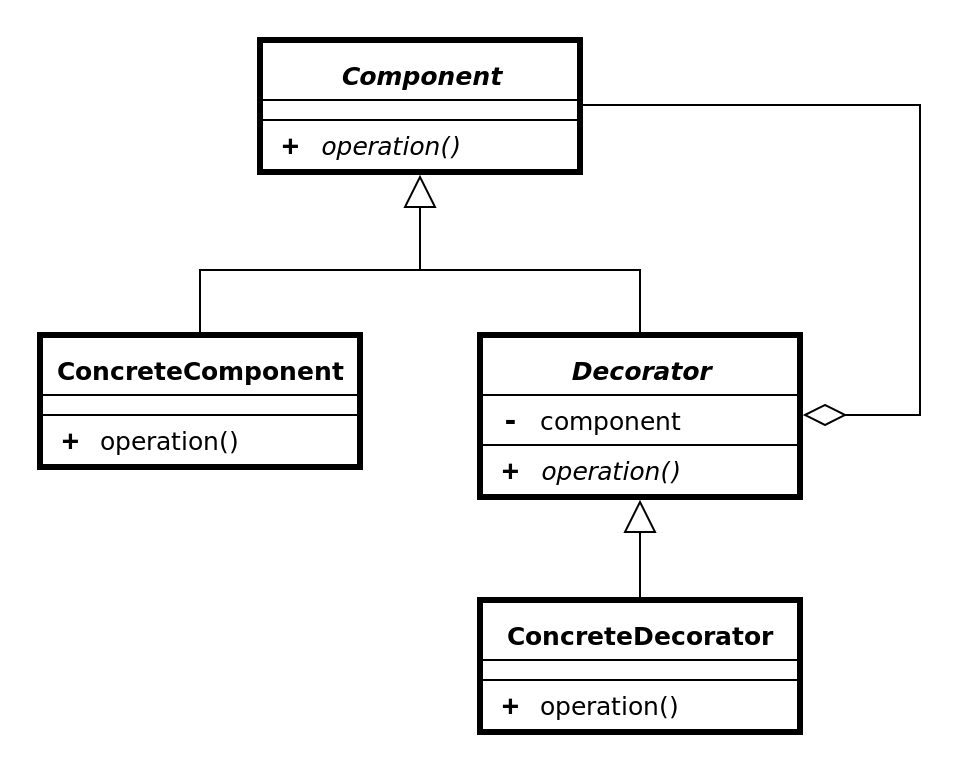
Purpose
A flexible mechanism for combining objects at runtime to extend functionality.
Implementation
abstract class Component
{
abstract public function Operation();
}
class ConcreteComponent extends Component
{
public function Operation()
{
return 'I am component';
}
}
abstract class Decorator extends Component
{
protected
$_component = null;
public function __construct(Component $component)
{
$this -> _component = $component;
}
protected function getComponent()
{
return $this -> _component;
}
public function Operation()
{
return $this -> getComponent() -> Operation();
}
}
class ConcreteDecoratorA extends Decorator
{
public function Operation()
{
return '<a>' . parent::Operation() . '</a>';
}
}
class ConcreteDecoratorB extends Decorator
{
public function Operation()
{
return '<strong>' . parent::Operation() . '</strong>';
}
}
// Example
$Element = new ConcreteComponent();
$ExtendedElement = new ConcreteDecoratorA($Element);
$SuperExtendedElement = new ConcreteDecoratorB($ExtendedElement);
The Facade Pattern
The facade pattern is a software-design pattern commonly used with object-oriented programming. Analogous to a facade in architecture, a facade is an object that serves as a front-facing interface masking more complex underlying or structural code.
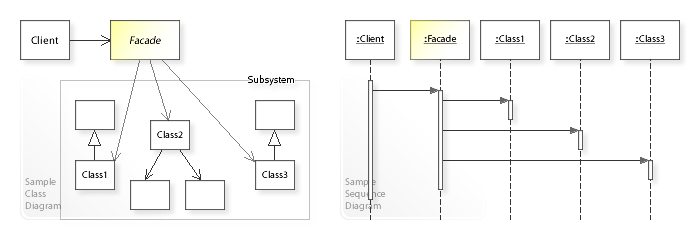
Purpose
Creating a simple interface to complex or variable systems.
Implementation
In this implementation, the creation and initialization of subsystems are hard wired into the code; this can be avoided by entering the factory pattern or using a parameterized constructor for the facade.
/**
* SystemA
*/
class Bank
{
public function OpenTransaction() {}
public function CloseTransaction() {}
public function transferMoney($amount) {}
}
/**
* SystemB
*/
class Client
{
public function OpenTransaction() {}
public function CloseTransaction() {}
public function transferMoney($amount) {}
}
/**
* SystemC
*/
class Log
{
public function logTransaction() {}
}
class Facade
{
public function transfer($amount)
{
$Bank = new Bank();
$Client = new Client();
$Log = new Log();
$Bank->OpenTransaction();
$Client->OpenTransaction();
$Log->logTransaction('Transaction open');
$Bank->transferMoney(-$amount);
$Log->logTransaction('Transfer money from bank');
$Client->transferMoney($amount);
$Log->logTransaction('Transfer money to client');
$Bank->CloseTransaction();
$Client->CloseTransaction();
$Log->logTransaction('Transaction close');
}
}
// Client code
$Transfer = new Facade();
$Transfer->transfer(1000);
Summary
In this post, I looked at a few of the ways that classes and objects can be organized in a system.
Marat Badykov
Full-stack web developer. I enjoy writing Php, Java, and Js.