ES6, also known as ECMAScript 2015, is a significant update to the language, and the first update to the language after ES5 was standardized in 2009.
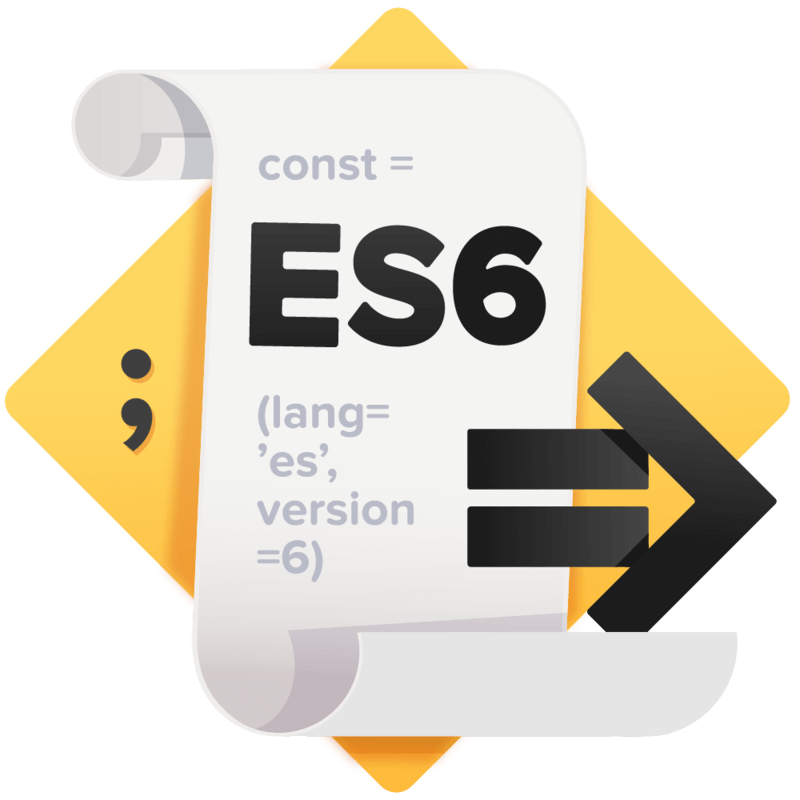
In these series of posts, I will try to cover the main changes that have been introduced in ES6.

Features
- let and const
- arrow functions
- template literals
- spread and rest operators
- destructuring arrays and objects
let and const
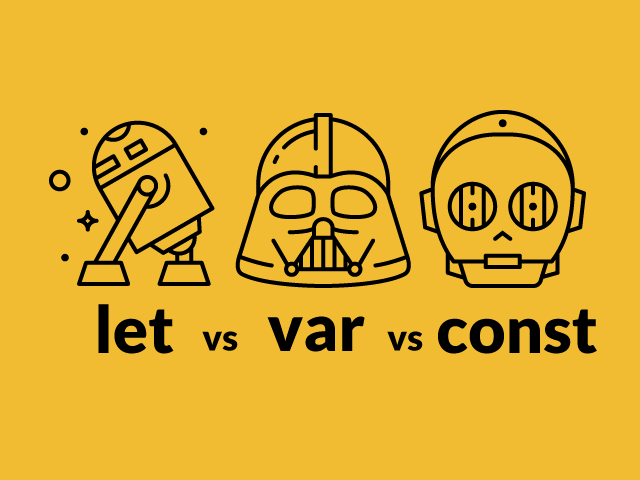
let and const keywords allows you to declare variables that are limited to the scope of a block statement, or expression on which it is used, unlike the var keyword, which declares a variable globally, or locally to an entire function regardless of block scope.
The const declaration creates a read-only reference to a value so it can not be reassigned.
Example
for (let i=0; i < array.length; i++) {
//some code
}
console.log(i) // Uncaught ReferenceError: i is not defined because of let is block-scoped
const variable = 5;
variable = 6; // Uncaught TypeError: Assignment to constant variable
Browser compatibility
const
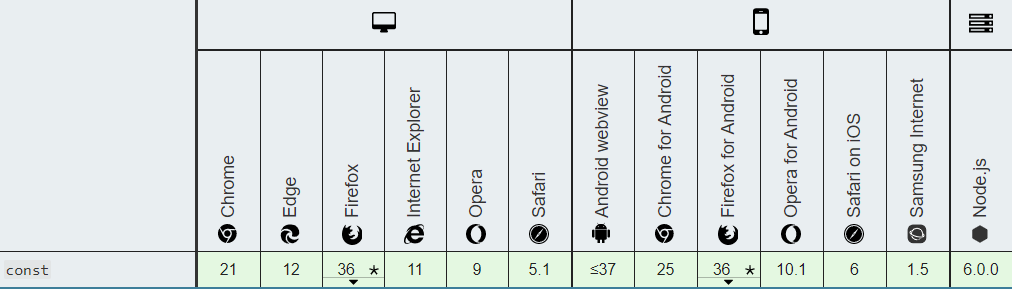
let
Arrow functions
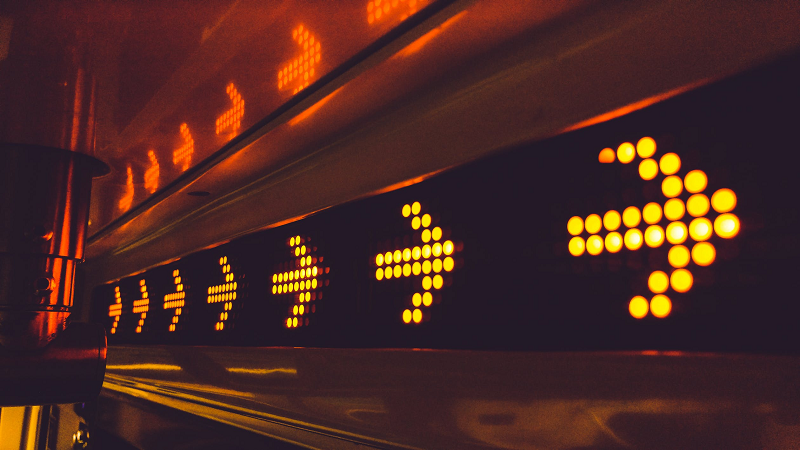
An arrow function expression is a compact alternative to a traditional function expression
Differences & Limitations:
- Does not have its own bindings to this or super, and should not be used as methods.
- Does not have arguments, or new.target keywords.
- Not suitable for call, apply and bind methods, which generally rely on establishing a scope.
- Can not be used as constructors.
- Can not use yield, within its body.
Example
// Traditional Function
function (a){
return a + 100;
}
// Arrow Function
a => a + 100;
Browser compatibility
Template literals
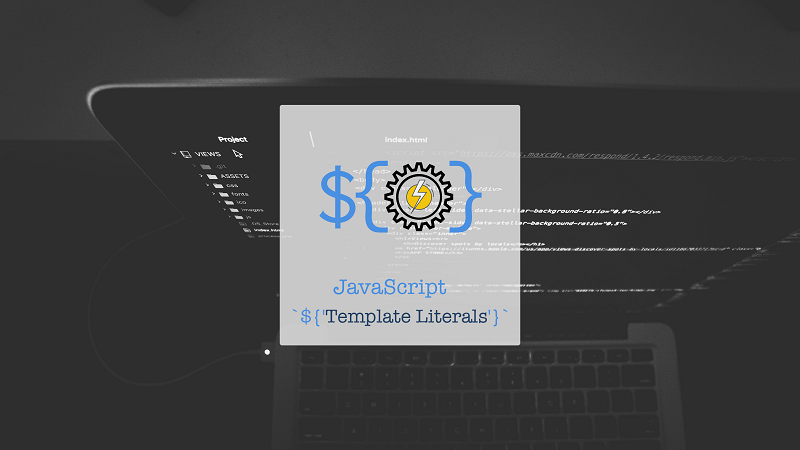
Template literals are string literals allowing embedded expressions. You can use multi-line strings and string interpolation features with them.
Example
// es5
function sayBuy(name){
return "Buy, " + name;
}
// es6 - template literals
function sayBuy(name){
return `Buy, ${name}`;
}
Browser compatibility
Spread and rest operators
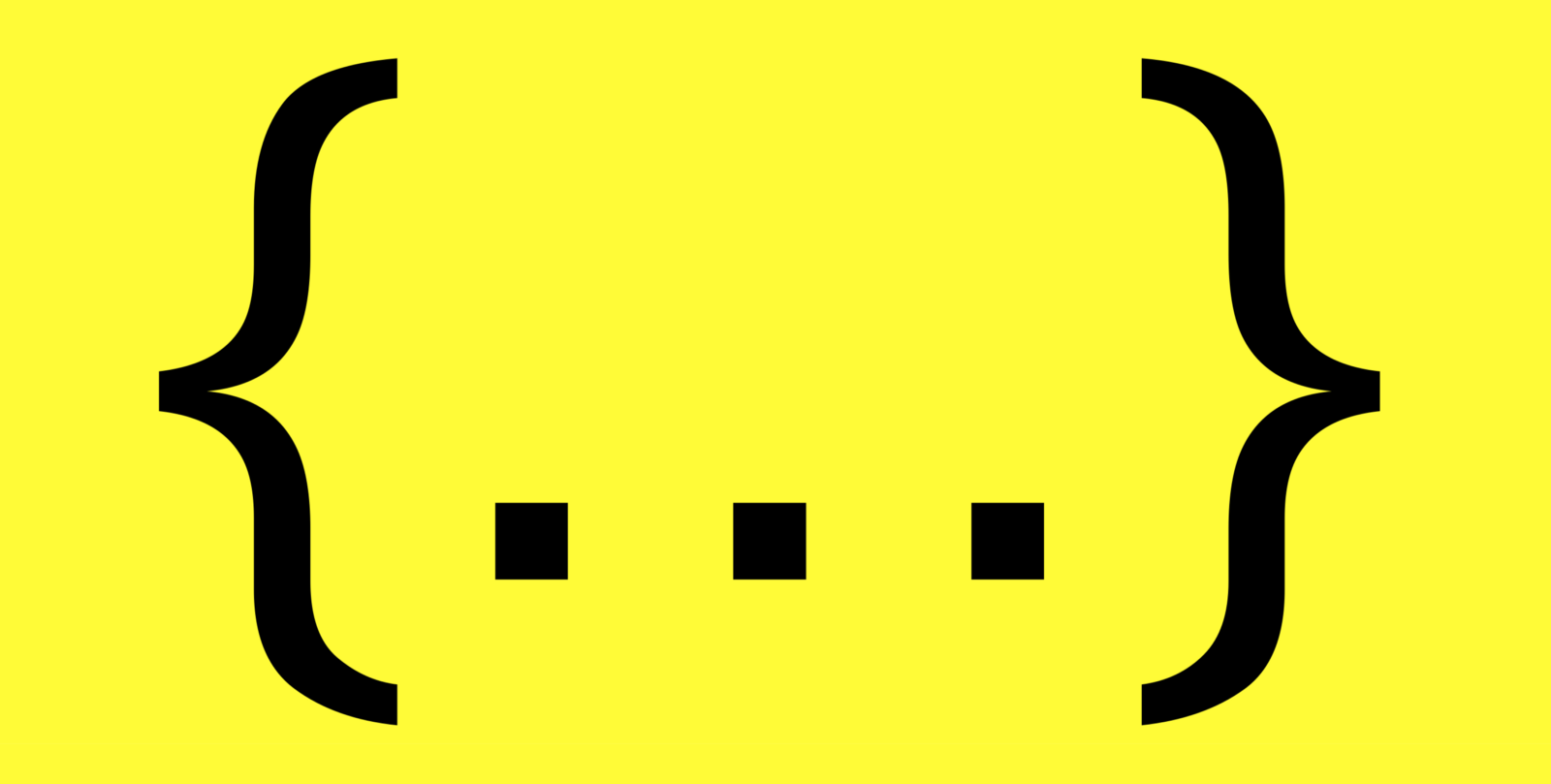
Spread syntax (…) allows an iterable such as an array expression or string to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected, or an object expression to be expanded in places where zero or more key-value pairs (for object literals) are expected.
Example
function sum(x, y, z) {
return x + y + z;
}
const numbers = [1, 2, 3];
console.log(sum(...numbers));
Rest syntax looks exactly like spread syntax. In a way, rest syntax is the opposite of spread syntax. Spread syntax “expands” an array into its elements, while rest syntax collects multiple elements and “condenses” them into a single element.
Example
function sum(x, y, ...z) {
let sum = x + y;
z.forEach(value => (sum += value);
return value;
}
console.log(sum(1, 2, 3, 4, 5));
Browser compatibility
Destructuring arrays and objects
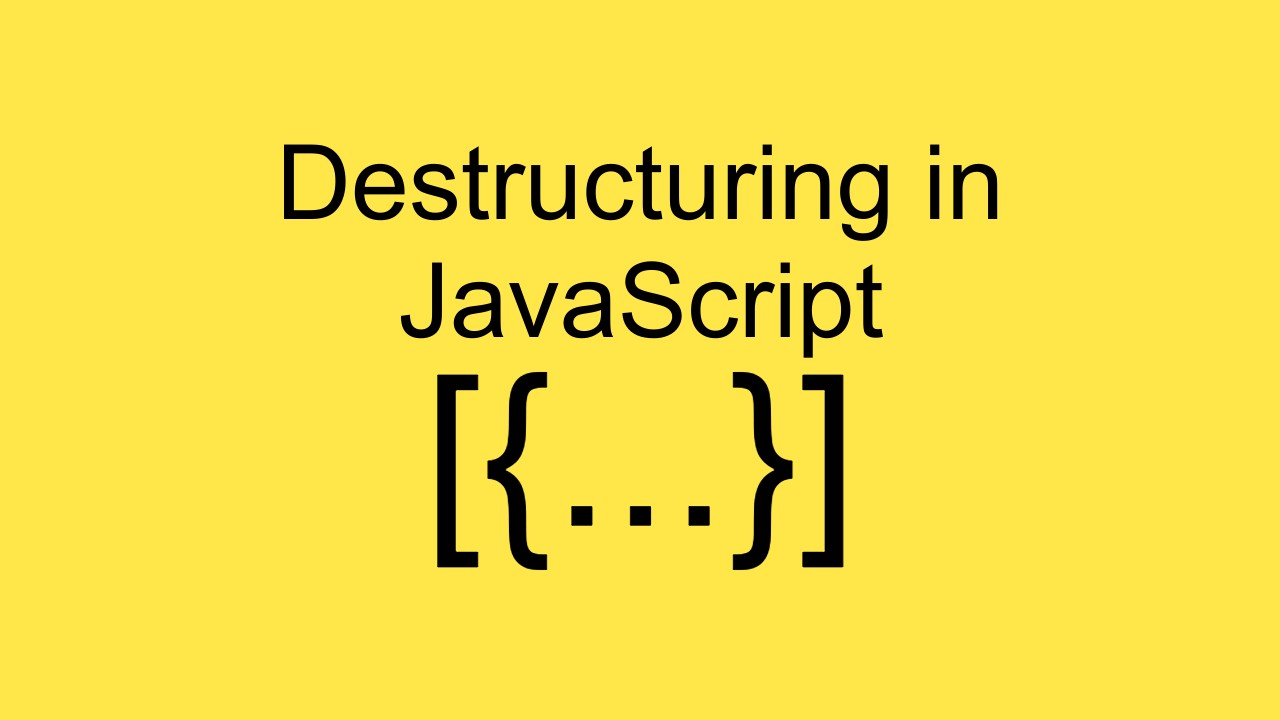
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
let a, b, rest;
[a, b] = [10, 20];
console.log(a); // 10
console.log(b); // 20
[a, b, ...rest] = [10, 20, 30, 40, 50];
console.log(a); // 10
console.log(b); // 20
console.log(rest); // [30, 40, 50]
({ a, b } = { a: 10, b: 20 });
console.log(a); // 10
console.log(b); // 20
// Stage 4(finished) proposal
({a, b, ...rest} = {a: 10, b: 20, c: 30, d: 40});
console.log(a); // 10
console.log(b); // 20
console.log(rest); // {c: 30, d: 40}
Browser compatibility
Summary
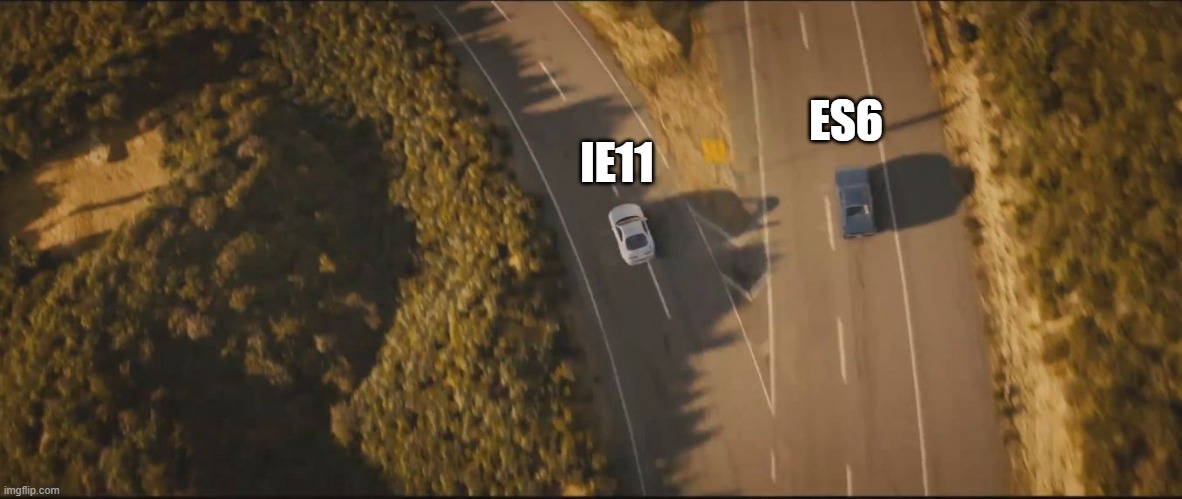
Marat Badykov
Full-stack web developer. I enjoy writing Php, Java, and Js.